はじめに
このスクリプトは、売上データをもとに 購入回数や売上合計を集計し、ピボットテーブルを作成する ためのものです。pandas の pivot_table()
を活用し、商品・顧客・地域ごとにデータを整理します。
pivot_table_maker.py
ソースコード
def mk_pivot_table(df, index_col="purchase_month"):
"""
指定したデータフレームから、購入月ごとに異なる指標でピボットテーブルを作成する関数。
Args:
df (pd.DataFrame): 分析対象のデータフレーム
index_col (str): ピボットテーブルのインデックスとする列(デフォルトは "purchase_month")
Returns:
tuple: (購入回数(商品ごと), 購入金額合計(商品ごと), 購入回数(顧客ごと), 購入回数(地域ごと))
"""
# 商品ごとの購入回数("size" により各商品の購入件数をカウント)
by_item = df.pivot_table(
index=index_col, columns="item_name", aggfunc="size", fill_value=0
)
# 商品ごとの売上合計("sum" により各商品の合計金額を計算)
by_price = df.pivot_table(
index=index_col,
columns="item_name",
values="item_price",
aggfunc="sum",
fill_value=0,
)
# 顧客ごとの購入回数(顧客名がデータに存在する場合のみ作成)
by_customer = (
df.pivot_table(index=index_col, columns="顧客名", aggfunc="size", fill_value=0)
if "顧客名" in df.columns
else None
)
# 地域ごとの購入回数(地域情報がデータに存在する場合のみ作成)
by_region = (
df.pivot_table(index=index_col, columns="地域", aggfunc="size", fill_value=0)
if "地域" in df.columns
else None
)
return by_item, by_price, by_customer, by_region
処理の概要
このスクリプトは以下の処理を行います。
- 商品ごとの購入回数の集計:
size
を使用し、各商品の購入件数をカウント。 - 商品ごとの売上合計の集計:
sum
を使用し、各商品の売上合計を計算。 - 顧客ごとの購入回数の集計:
size
を使用し、顧客ごとの購入件数をカウント(顧客情報が存在する場合)。 - 地域ごとの購入回数の集計:
size
を使用し、地域ごとの購入件数をカウント(地域情報が存在する場合)。
ピボットテーブルの作成
mk_pivot_table()
は、売上データを集計し、異なる指標ごとにピボットテーブルを作成します。
def mk_pivot_table(df, index_col="purchase_month"):
"""
指定したデータフレームから、購入月ごとに異なる指標でピボットテーブルを作成する関数。
Args:
df (pd.DataFrame): 分析対象のデータフレーム
index_col (str): ピボットテーブルのインデックスとする列(デフォルトは "purchase_month")
Returns:
tuple: (購入回数(商品ごと), 購入金額合計(商品ごと), 購入回数(顧客ごと), 購入回数(地域ごと))
"""
# 商品ごとの購入回数("size" により各商品の購入件数をカウント)
by_item = df.pivot_table(
index=index_col, columns="item_name", aggfunc="size", fill_value=0
)
# 商品ごとの売上合計("sum" により各商品の合計金額を計算)
by_price = df.pivot_table(
index=index_col,
columns="item_name",
values="item_price",
aggfunc="sum",
fill_value=0,
)
# 顧客ごとの購入回数(顧客名がデータに存在する場合のみ作成)
by_customer = (
df.pivot_table(index=index_col, columns="顧客名", aggfunc="size", fill_value=0)
if "顧客名" in df.columns
else None
)
# 地域ごとの購入回数(地域情報がデータに存在する場合のみ作成)
by_region = (
df.pivot_table(index=index_col, columns="地域", aggfunc="size", fill_value=0)
if "地域" in df.columns
else None
)
return by_item, by_price, by_customer, by_region
pivot_table()
を使用し、各指標ごとにデータを集計。- 商品ごとの購入回数 (
by_item
)、商品ごとの売上合計 (by_price
)、顧客ごとの購入回数 (by_customer
)、地域ごとの購入回数 (by_region
) をそれぞれ作成。 - 顧客名や地域情報がデータに存在しない場合は
None
を返す。
このスクリプトを活用することで、売上データを購入回数や売上金額ごとに集計し、分析をスムーズに行うことができます。
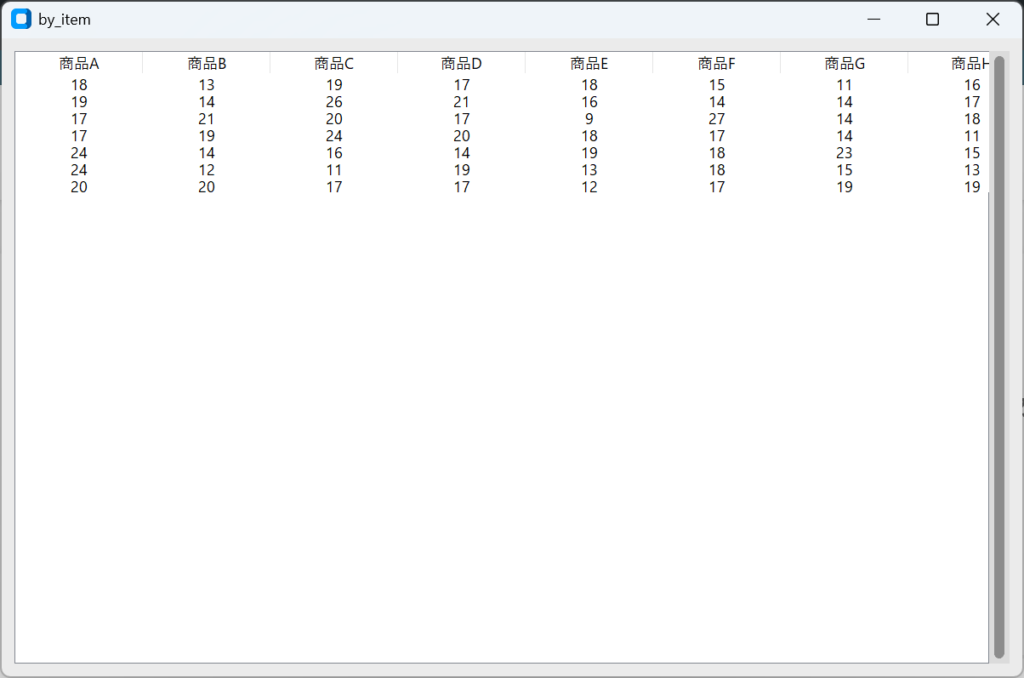
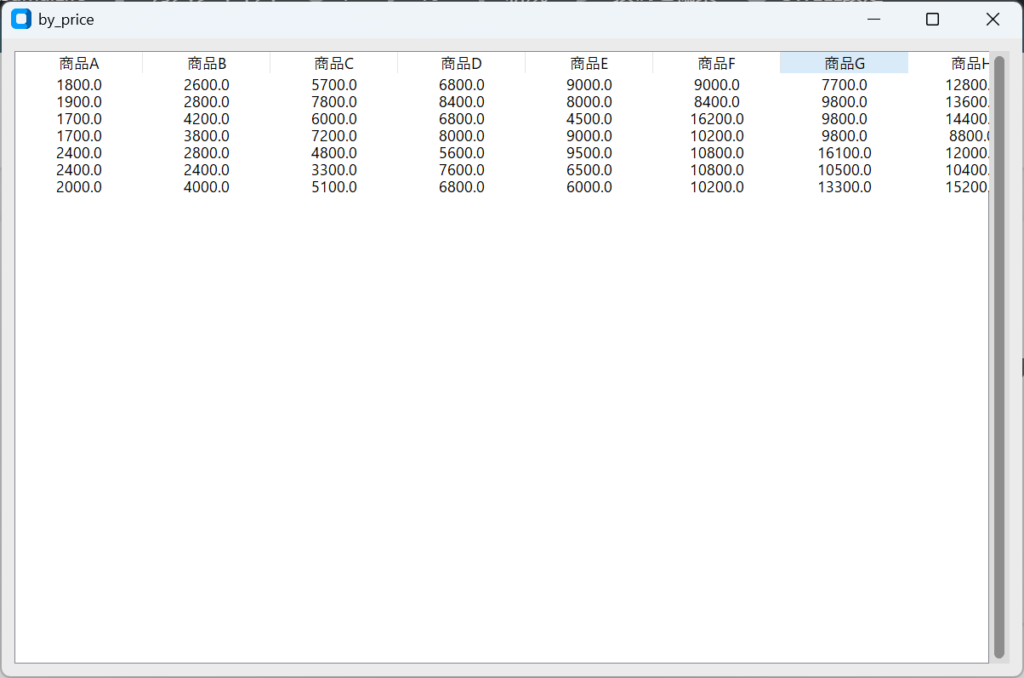
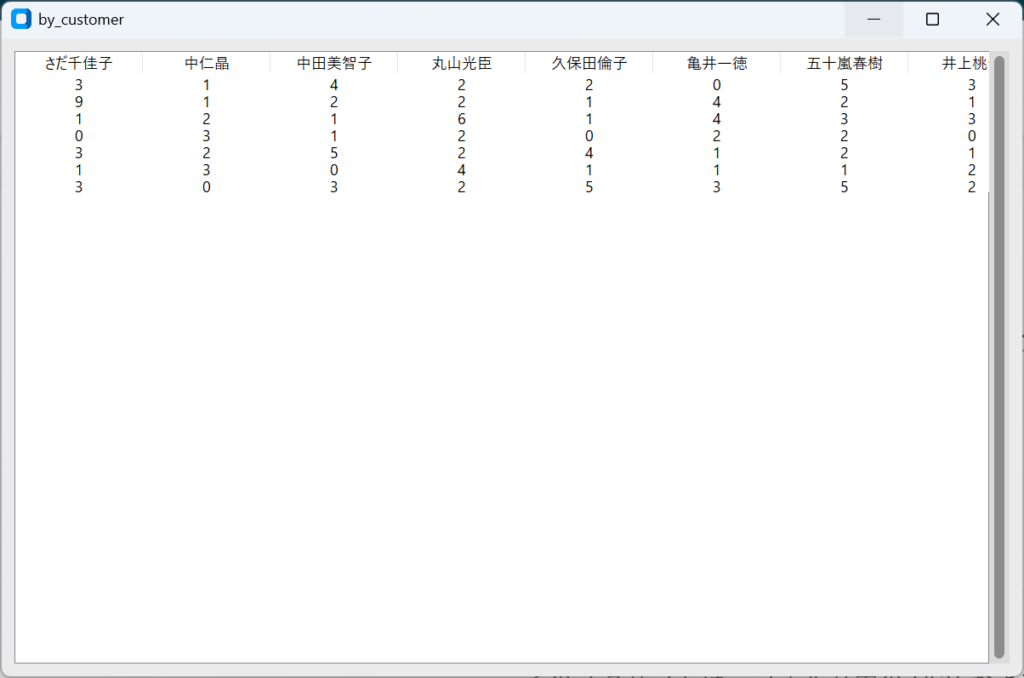
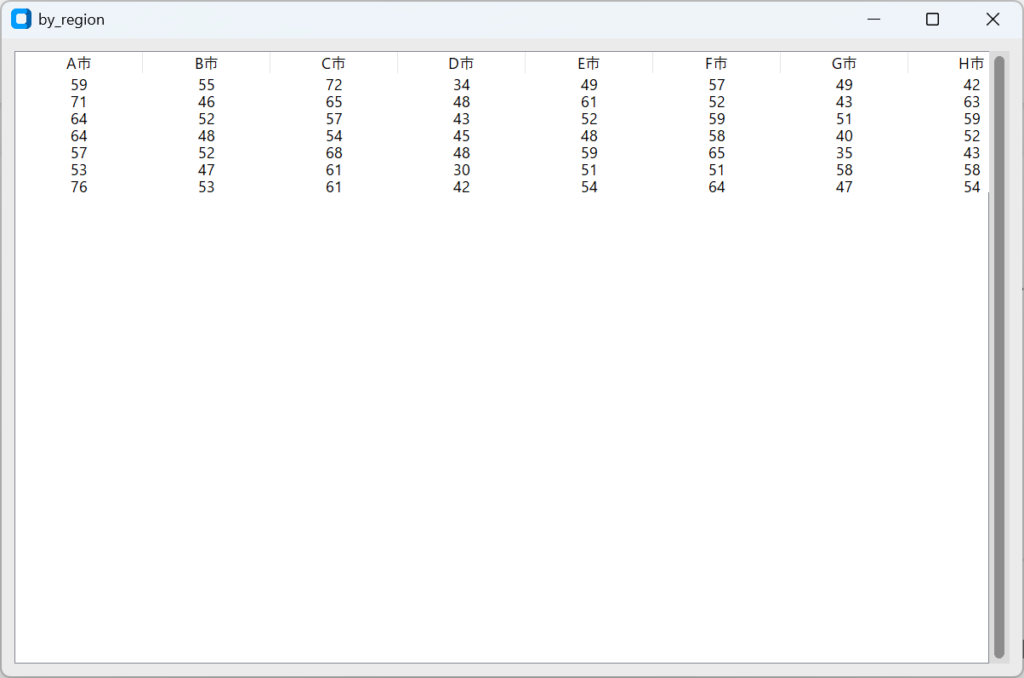
コメント